28 Oct 2015
This post is mainly a non-exhaustive list of things that could go wrong when you deploy you app.
1. You are not listening on the correct port
In most cases, services like Heroku will make you use a specific port. And in most cases it is
not 8080, 8000 or 3000. This can be taken into account in just one line of code:
becomes
var port = process.env.PORT || 8080;
The process.env.PORT will ensure that the correct port will be set when we deploy.
2. Your local npm and node versions are different from those installed on the server
To force the server to install the versions you want you can add the following to your package.json
:
"engines": {
"node": "0.12.7",
"npm": "2.14.2"
}
These are the versions that I want to install on the server, because these are the ones I use locally.
To figure out which version you need to put in, type this in you terminal window:
npm -v
and node -v
3. Check your .gitignore when pushing to your server remote
A lot of deployment services make you push to a remote to deploy. This one took 6 hours out of my day to figure out! Do better than I did.
4. Static files should be in your server folder
Your app should be scaffolded like so:
App
--Server
----Public
------index.html
------css
------img
----index.js
Where index.js is the script that starts up your server.
5. Have an npm start script or Procfile to start your server
Easiest way to ensure that your server actually executes your server code is to include the following in your package.json:
"scripts": {
"start": "node server/index.js"
}
6. A word of encouragement
via GIPHY
You are not alone in your suffering. Deployment is HARD. You will probably waste multiple hours on minor issues that do not learn you a lot about deployment. Everybody who learns this stuff has a HARD time at first.
28 Sep 2015
I wanted to make something that gives you a higher order view
of how different Wikipedia pages are linked together. I made this website during my time at Hackreactor. Since I only had two days, I had to zone in on a particular type of page. Since I’ve always had a hard time visualizing the link between monarchs and their successors, that is exactly what I built!
Type in “Henry VIII” on Wikigraph:
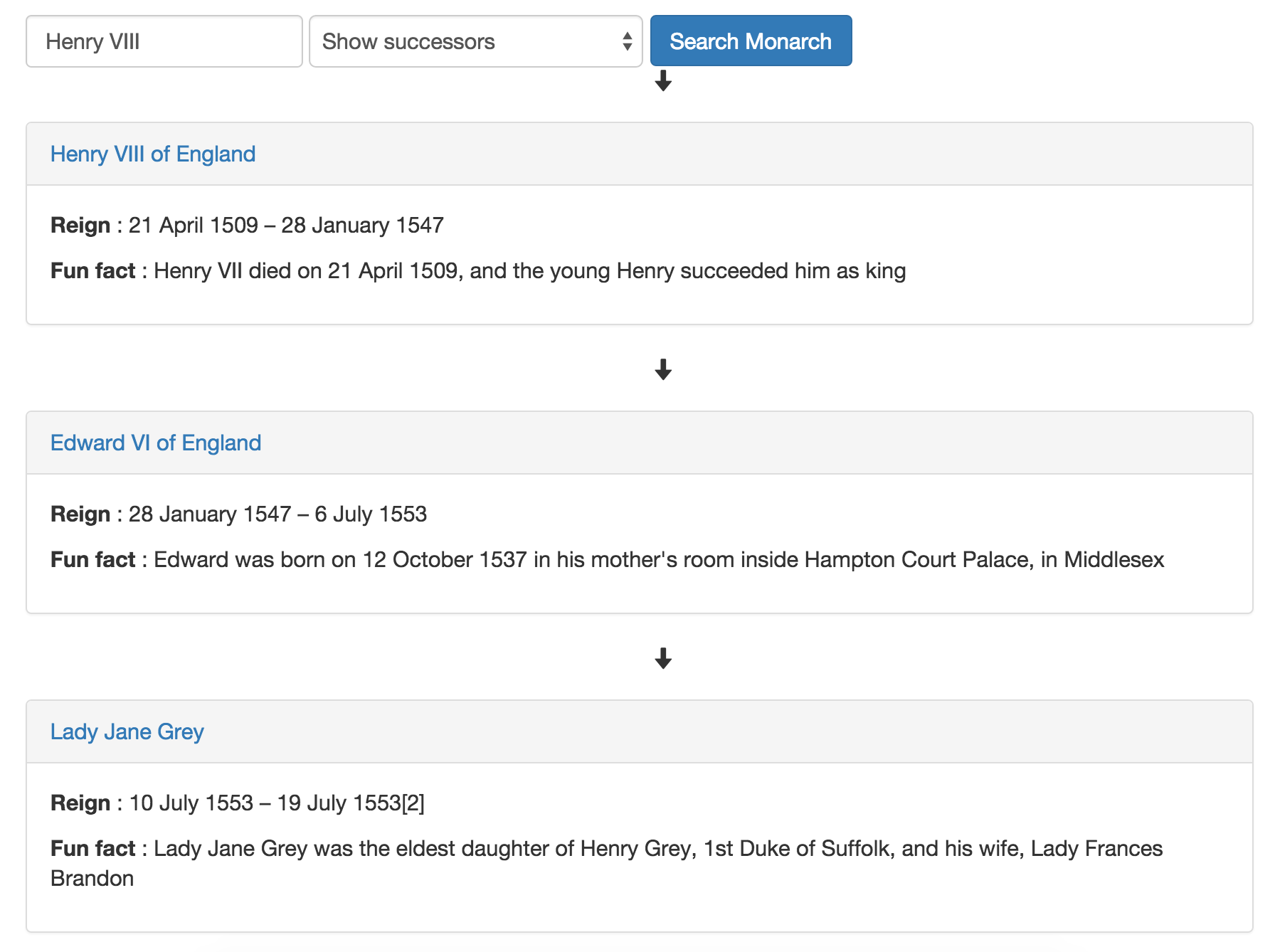
How it works (Wikipedia API + JQuery + d3):
Wiki-graph uses Wikipedia’s search API. Once it has found the page, it reads the HTML (via an ajax call) and looks for the “successor” field in the infobox. This piece of text will point to another wikipedia page. Wikigraph will then make an ajax call to the successor’s page. You could look at it as a recursive ajax call. At the end of every ajax call, we pass down the data to d3. This allows us to render something for the user while we are fetching the extra data. The original goal of using d3 was to render some kind of graph visualization (which was out of reach for the time I was given).
28 Sep 2015
Use console.table
to print nested objects and arrays to the console.
var me = [
{
firstname: "Tim",
lastname: "Scheys"
},
{
firstname: "Keith",
lastname: "Anderson"
},
{
firstname: "John",
lastname: "Yung"
}
];
console.log(me);
console.table(me);
var fruits = [
["apple", "orange", "banana"],
["peach", "pear", "melon"]
];
console.log(fruits);
console.table(fruits);
The above code will result in the following console messages:
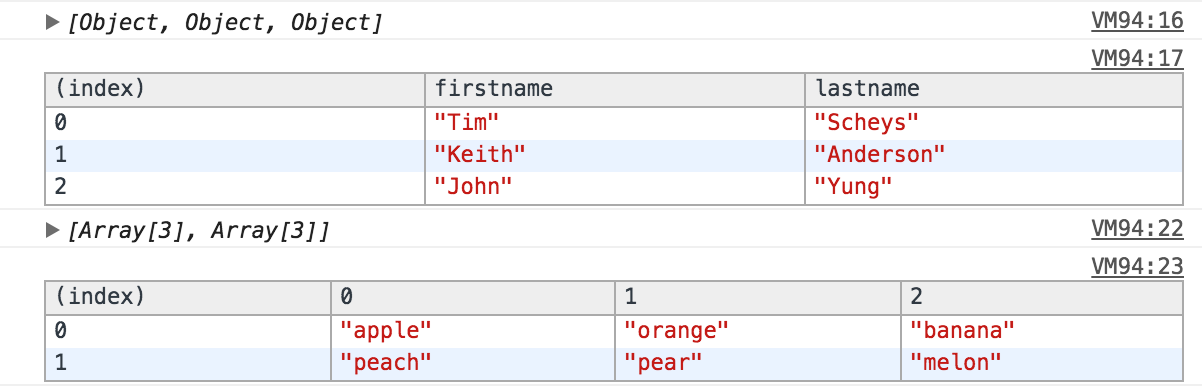
As you can see this is approximately 100 times better than a standard console.log
This works in Chrome, Firefox and Opera.
28 Sep 2015
Use the native forEach function instead.
Example:
var numbers = [1,9,9,2];
for(var i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
// is equivalent to
numbers.forEach(function(value, index, array) {
/*via this callback function
you still have access to the whole array
and the index of the current value.*/
console.log(value);
});
Advantages:
.forEach()
is easier to understand for a noncoder.
- Faster to type.